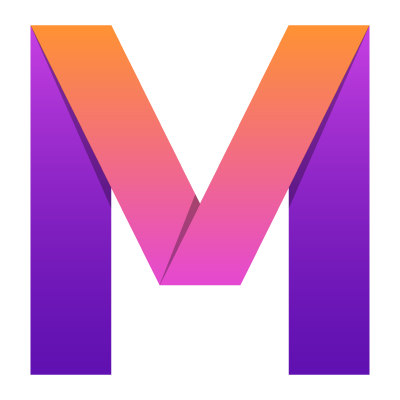
6. Applying Layouts
How to apply the layouts created with MobileUI on Android and iOS.
MobileUI brings a cross-platform layout system to Android and iOS. To apply MobileUI layouts, you need some lines of code on both platforms. The following sections describe the integration of the layout system on Android and iOS.
Hint: When you create a new MobileUI app project in Android Studio, the generated source already applies the first layout to an Android Activity and an iOS UIViewController as described below. You are free to change this as required.
The concept of bindingContext
The MobileUI layout system uses UI data binding to connect logic written in Java or Kotlin with the user interface declared in XML. The bindingContext is an arbitrary Java object that is connected with the layout to function as logic implementation and data provider for the latter. With this architecture, MobileUI implements the MVVM pattern. Therefore, the bindingContext may be referred to as View Model.
In the following sections, we discuss different options for applying the bindingContext. When you are developing a cross-platform app as generated by the MobileUI plugin for Android Studio, it is convention to use an object from the app-commons module as bindingContext to enable code-reuse.
Using MobileUI Layouts in your Android app
The MobileUI Framework for Android comes with the class io.nevernull.mobileui.android.MobileUILayout
. You can use an instance of MobileUILayout
as content view for your Activities and Fragments. The MobileUILayout
(like any other Android View) requires an Android Context as first parameter. The second parameter references the XML-layout to be displayed. The third parameter is the bindingContext for your layout.
new MobileUILayout(this, "Main.layout.xml", bindingContext);
MobileUILayouts in Activities
To use the MobileUILayout as UI for an Activity, set it as content view in the Activity's onCreate()
method.
You can either use the Activity as binding context, so that you need to create properties and action callbacks here:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new MobileUILayout(this, "Main.layout.xml", this));}
Alternatively, you can bind any other object - e.g. from your app-common module - to reuse code between Android and iOS like shown in the following example:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
MainController mainController = new MainController(); setContentView(new MobileUILayout(this, "Main.layout.xml", mainController));}
The latter is the default, when you create a new MobileUI app with our MobileUI Plugin for Android Studio.
MobileUILayouts in Fragments
Modern Android apps work with Fragments. We recommend to build your app with Android's Navigation component, which simplifies the use for Fragments significantly.
To use a MobileUILayout as UI for your Fragment, create and return it in the Fragment's onCreateView()
method. In the following example, the binding context is the Fragment, meaning properties and
callbacks must be implemented here.
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return new MobileUILayout(this, "Main.layout.xml", this);}
Alternatively, bind to any other object. This is typically done with a class from your app-common module as shown below:
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
MainController mainController = new MainController(); return new MobileUILayout(this, "Main.layout.xml", mainController);}
Using MobileUI Layouts in your iOS app
The MobileUI Framework for iOS contains the class io.nevernull.mobileui.ios.MobileUILayout
. You can use a MobileUILayout
as view for any UIViewController by setting it with the setView()
method during the loadView()
method call.
The first parameter of the MobileUILayout's constructor must be the current UIViewController. The second parameter
is the name of the XML layout file in your assets folder. The third parameter is the binding context.
You can use the UIViewController as binding context by referring to this
as shown in the following example.
@Override
public void loadView() {
setView(new MobileUILayout(this, "Main.layout.xml", this));
}
Alternatively, you can bind any other object. We recommend to create a custom controller that resides in your app-common module and can be reused from Android and iOS as shown here:
@Override
public void loadView() {
MainController mainController = new MainController();
setView(new MobileUILayout(this, "Main.layout.xml", mainController));
}
The latter is the default, when you create a new MobileUI app with our MobileUI Plugin for Android Studio.