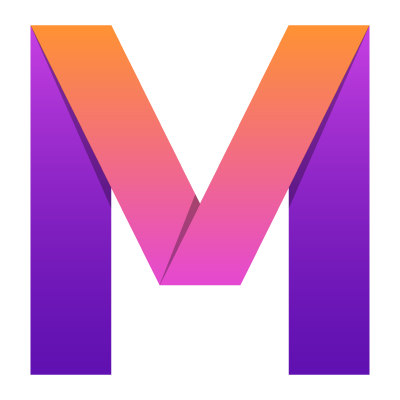
🔌 Internationalization (i18n)
Supporting different languages in your UI and logic
The i18n Plugin PURPLE makes Java's internationalization APIs easily accessible in your MobileUI applications. You can use messages from Java ResourceBundles
within your MobileUI Layouts and easily inject a Messages
object into your business logic.
Plugin Infos | |
---|---|
Dependency | "io.nevernull:mobileui-plugin-i18n" |
Api Docs | io.nevernull.mobileui.i18n |
Main Interface | io.nevernull.mobileui.i18n.Messages |
Translation with ResourceBundles
MobileUI leverages Java's standard internationalization support to let you translate your user interfaces and runtime messaging to the user. Under the hood, it loads Java ResourceBundles from a properties file. For now, the plugin expects the resource bundle messages
to be present within the folder app-common/src/main/resources/
. This means, you can place one or several files with the scheme messages_[locale-id].properties
in this folder where [locale-id]
is the ID of the language, you are providing. The following listings exemplify such a resource bundle that comes with a default file (no locale) and a German translation:
simpleMessage=MobileUI rocks internationally!
messageWithArguments=Test with {0} good arguments
messageWithFormattedArguments=At {1,time} on {1,date,long}, there was {2} on planet {0,number,integer}.
simpleMessage=MobileUI rockt international!
messageWithArguments=Test mit {0} guten Argumenten
messageWithFormattedArguments=Um {1,time} am {1,date,long} fand auf Planet Nummer {0,number,integer} {2} statt.
The great thing about ResourceBundles is, that Android Studio comes with a built-in editor for these files. Use this approach to create translations for your apps easily:
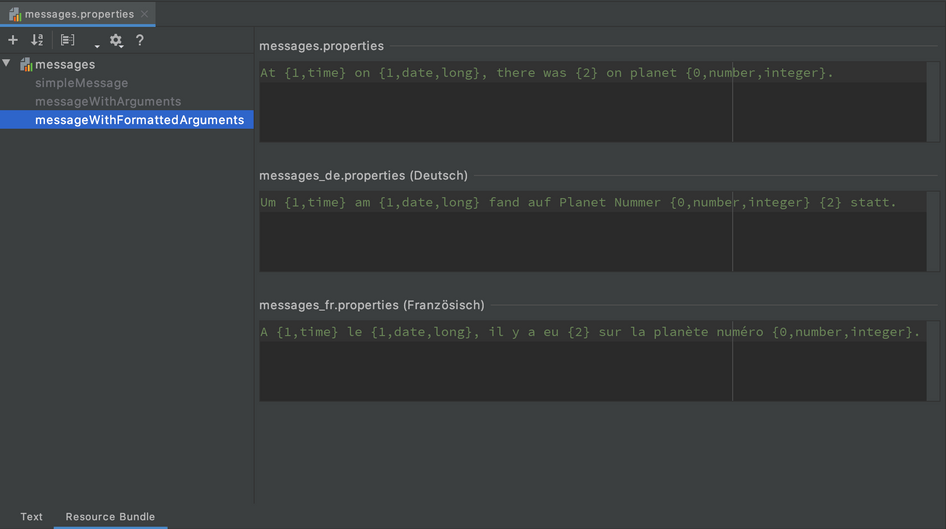
Important: The resource files of the
app-common
module are sometimes not copied correctly by the RoboVM IDEA plugin. Please follow the RoboVM Resources Workaround to make sure, the iOS app has all required resources.
Use Messages in your layouts
With activated i18n plugin, the MobileUI template engine automatically adds the object messages
to your template. The object is an instance of the Messages
interface, so that you have the same methods for looking up messages as with Java and Kotlin.
Note: The messages object is integrated into MobileUI's advanced layout caching mechanism. Whenever the device's locale changes, the MobileUI Framework will automatically re-render the layout. If you need more dynamic message creation, please use runtime bindings as discussed below.
<Layout xmlns="urn:nevernull:mobileui:layout">
<LinearLayout orientation="vertical">
<TextView text="@{messages.get('simpleMessage')}"/>
<TextView text="@{messages.get('messageWithArguments', 42)}"/>
<TextView text="@{messages.get('messageWithFormattedArguments', 7, new Date(), 'Party')}"/>
<!-- Use runtime bindings to have dynamic message creation, see below -->
<TextView text="#{messageWithFormattedArguments}"/>
</LinearLayout>
</Layout>
Use Messages in your Logic
Use a Messages
object in your Java or Kotlin code to translate strings. In a managed object, you typically use constructor injection to get hold of an instance as shown below:
import io.micronaut.context.annotation.Prototype;
import io.nevernull.mobileui.i18n.Messages;
@Prototype
public class MainController {
public String simpleMessage;
public String messageWithArguments;
public String messageWithFormattedArguments;
public I18nController(Messages messages) {
simpleMessage = messages.getOrDefault("simpleMessage", "This would be the default text.");
messageWithArguments = messages.get("messageWithArguments", 42);
messageWithFormattedArguments = messages.get("messageWithFormattedArguments", 7, new Date(), "Party");
}
}