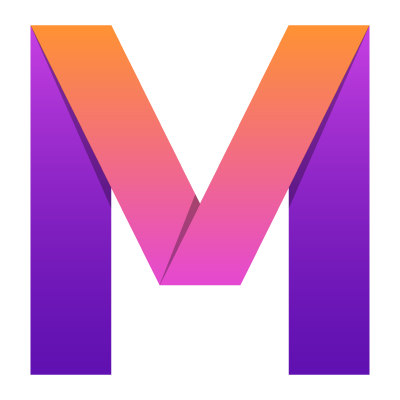
LifecycleAware Controllers
Learn how to make your your bindingObjects take part in the UI lifecycle
Your bindingObjects can implement lifecycle callbacks that work for Android and iOS in the same way.
Applying the LifecycleAware interface
To apply lifecycles to your bindingObjects:
- Implement the
io.nevernull.mobileui.lifecycle.LifecycleAware
interface with your class. - Override one or more of the lifecycle methods given by the interface. As these methods are interface default methods, you are free to choose from the list. You are not required to implement all of them.
- Make sure, you are using the correct constructors for your
MobileUILayout
s on both platforms- On Android, use the constructor taking the Activity as first attribute, if you want to use the Activity's lifecycle. If you are using a Fragment, use the corresponding constructor with the Fragment as first attribute.
- On iOS make sure, you are passing the UIViewController as first argument to MobileUILayout's constructor. The lifecycle will be the one of the UIViewController.
An example for a LifecycleAware controller in your app-common
module is shown below. The controller only implements the onStart
and onStop
methods to make some simple logging:
import io.nevernull.mobileui.lifecycle.LifecycleAware;
public class MyController implements LifecycleAware<Object> {
@Override
public void onStart(Object o) {
System.out.println("Started");
}
@Override
public void onStop(Object o) {
System.out.println("Stopped");
}
}
Lifecycle Mappings
The following table shows the mapping from the LifecycleAware
methods to the native lifecycle methods. This is useful for cases where you want to combine native code with the LifecycleAware functionality of MobileUI:
MobileUI LifecycleAware | Android Activity & Fragment | iOS UIViewController |
---|---|---|
onCreate | onCreate | viewDidLoad |
š„Bindings are fired | ||
onStart | onStart | viewWillAppear |
onResume | onResume | viewWillAppear |
onPause | onPause | viewWillDisappear |
onStop | onStop | viewDidDisappear |
onDestroy | onDestroy | doDispose |
Lifecycle and Bindings
As shown in the table above, their is a time in the lifecyle, when the bindings are fired by MobileUI to update the UI from the data in your controller. This takes place after the onCreate()
method has finished. This means, you can initialize your controller in the onCreate() method and don't have to care about firing all changes. The following code snippet exemplifies this approach:
public class MyController implements LifecycleAware<Object> {
public String greeting = "Start with NeverNullĀ® MobileUI today.";
@Override
public void onCreate(Object o) {
greeting = "Just created";
// No need to fire changes }
@Override
public void onStart(Object o) {
greeting = "Just started";
// Firing changes is required MobileUI.firePropertyChanged(this, "greeting"); }
}